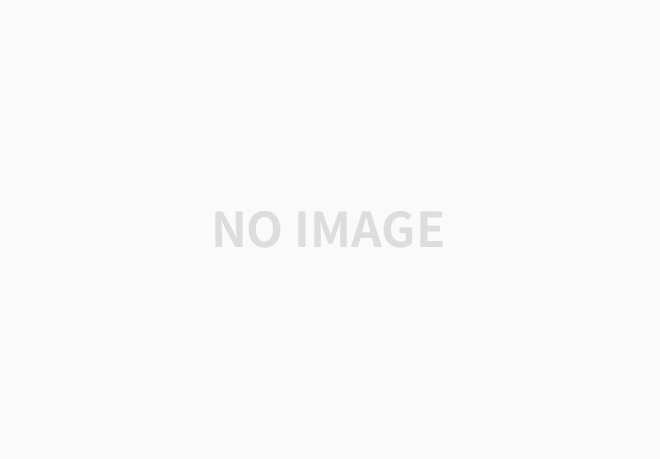
안드로이드 스튜디오의 xml의 기본적인 버튼과 폰트에 대한 코드를 얼추 여기에 정리하겠다.
(복습용으로 대충 썼다)
🎨 1. 버튼 (Button) 스타일링
<Button
android:layout_width="200dp" <!-- 버튼의 너비 -->
android:layout_height="wrap_content" <!-- 버튼의 높이 -->
android:text="클릭하세요" <!-- 버튼 내부 텍스트 -->
android:textSize="18sp" <!-- 버튼 글자 크기 -->
android:textColor="#FFFFFF" <!-- 버튼 글자 색상 -->
android:background="@color/blue" <!-- 버튼 배경 색상 -->
android:padding="10dp" <!-- 내부 여백 -->
android:gravity="center" <!-- 글자 중앙 정렬 -->
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"/>
- layout_width, layout_height → 크기 조절
- textSize, textColor → 글자 크기 및 색상
- background → 버튼 색상 변경
- gravity → 내부 텍스트 정렬
📏 2. 크기 관련 속성
android:layout_width="match_parent" <!-- 부모 크기만큼 차지 -->
android:layout_width="wrap_content" <!-- 내용 크기만큼만 차지 -->
android:layout_width="200dp" <!-- 200dp 크기로 고정 -->
- match_parent → 부모 크기만큼 확장
- wrap_content → 내용물만큼 크기 조절
- 고정 값 (ex: 200dp) → 직접 지정
🎭 3. 정렬 (중앙 정렬, 수평/수직 정렬)
android:gravity="center" <!-- 내부 요소(텍스트) 중앙 정렬 -->
android:gravity="start|center_vertical" <!-- 왼쪽 정렬 + 수직 중앙 -->
- gravity → 내부 컨텐츠 위치 조정
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintEnd_toEndOf="parent"
- ConstraintLayout에서 중앙 정렬
📌 4. 수평/수직 배치 (LinearLayout)
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical" <!-- 세로 방향 나열 -->
android:gravity="center_horizontal"> <!-- 중앙 정렬 -->
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="버튼 1"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="버튼 2"/>
</LinearLayout>
- android:orientation="vertical" → 세로 방향 정렬
- android:orientation="horizontal" → 가로 방향 정렬
🏗 5. 여백 & 패딩 (Margin, Padding)
android:padding="10dp" <!-- 내부 여백 (테두리와 내용 사이 간격) -->
android:layout_margin="10dp" <!-- 외부 여백 (다른 요소와의 거리) -->
- padding → 내부 요소와의 거리
- margin → 바깥 요소와의 거리
📌 텍스트 관련 XML 코드 정리
1️⃣ 기본 텍스트 뷰 (TextView)
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="안녕하세요!"
android:textSize="20sp"
android:textColor="#FF5733"
android:textStyle="bold"
android:gravity="center"
android:padding="10dp"
/>
🔹 속성 설명
- android:text="안녕하세요!" → 표시할 텍스트
- android:textSize="20sp" → 글자 크기 (sp 단위)
- android:textColor="#FF5733" → 글자 색상
- android:textStyle="bold" → 글자 스타일 (bold, italic, normal)
- android:gravity="center" → 텍스트 정렬
- android:padding="10dp" → 내부 여백
2️⃣ 텍스트 정렬 (Gravity & Layout Gravity)
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="가운데 정렬된 텍스트"
android:gravity="center"
/>
📌 Gravity vs Layout Gravity 차이
- android:gravity="center" → 텍스트 내용 중앙 정렬
- android:layout_gravity="center" → 뷰 자체를 중앙 정렬
3️⃣ 다양한 텍스트 스타일
<TextView
android:text="기본 텍스트"
android:textStyle="normal" />
<TextView
android:text="굵은 텍스트"
android:textStyle="bold" />
<TextView
android:text="기울임 텍스트"
android:textStyle="italic" />
<TextView
android:text="굵고 기울임 텍스트"
android:textStyle="bold|italic" />
🔹 android:textStyle 값:
- normal → 기본
- bold → 굵게
- italic → 기울임
- bold|italic → 굵고 기울임
4️⃣ 여러 줄 텍스트 & 자동 줄 바꿈
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="이것은 여러 줄로 표시되는 텍스트입니다. 길이가 길면 자동으로 줄 바꿈이 됩니다."
android:maxLines="3"
android:ellipsize="end"
android:lineSpacingExtra="4dp"
/>
🔹 속성 설명
- android:maxLines="3" → 최대 3줄까지만 표시
- android:ellipsize="end" → 글이 길면 ... 으로 생략
- android:lineSpacingExtra="4dp" → 줄 간격 추가
5️⃣ HTML 태그 적용
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/html_text"
android:autoLink="web"
/>
🔹 android:autoLink="web" → URL 자동 링크 적용
🔹 android:text="@string/html_text" → HTML 적용 가능
<string name="html_text"><![CDATA[<b>굵은 글자</b> <i>기울임</i> <font color='#FF0000'>빨간색</font>]]></string>
6️⃣ 클릭 가능한 텍스트
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="클릭하세요!"
android:textColor="@color/blue"
android:clickable="true"
android:onClick="onTextClick"
/>
🔹 Activity에서 클릭 이벤트 처리 (Java/Kotlin)
fun onTextClick(view: View) {
Toast.makeText(this, "텍스트 클릭됨!", Toast.LENGTH_SHORT).show()
}
✅ 정리
- 기본 텍스트 속성 → textSize, textColor, textStyle
- 정렬 → gravity, layout_gravity
- 줄 바꿈 & 생략 → maxLines, ellipsize
- HTML 적용 → <b>, <i>, <font>
- 클릭 가능 텍스트 → clickable, onClick
'개발 이모저모' 카테고리의 다른 글
[VSCode]한글이 깨져 보이는 파일 인코딩 변경하기 (0) | 2025.03.27 |
---|---|
커서 ai로 코딩하기. (2) | 2025.03.27 |
SSR 과 CSR (서버 사이드 렌더링/클라이언트 사이드 렌더링) (2) | 2025.03.20 |
vscode에서 쓰이는 유용한 기능 소개 (0) | 2025.03.13 |
카카오톡에 챗봇 연결하기 (0) | 2025.03.07 |